When communicating with humans, you can communicate through language (spoken and written), hand gestures, body language, and even facial expressions. These communication methods allow us to express our thoughts, needs, and ideas. Similarly, our interactions with computers, apps, and websites happen through interface (UI) components like screens, menus, and buttons or programming languages.
Behind the scenes, however, software elements communicate differently with each other. They don’t require a graphical user interface to interact. Instead, software elements use machine-readable interfaces called Application Programming Interfaces (APIs) to communicate and exchange data.
APIs let different software systems talk to each other and share data through simple commands called API calls. The data exchanged between these systems is formatted in a standardized way, with JSON being the most common format.
You might not realize it, but APIs are everywhere, and they’re extensively used in software development — particularly software integrations. With Glide’s APIs, you can connect almost any tool or integration. The possibilities are endless. You can connect your CRM with your email marketing software, accounting software, customer support platforms, and more.
Even if you’re not an engineer, it’s still a good idea to get familiar with APIs and the basics of how they operate. Using APIs can simplify your workflows by automating tasks and requests and gives you control over your own tools.
If you aren't already familiar with technology like remote procedure call (RPC) and REST APIs, you will be by the end of this guide. You'll also be perfectly set up to tackle Glide's Level 3 Certification.
What is an API
An API is a collection of programming code that enables two software components to exchange data and communicate with each other. It also contains the terms of this data exchange. Think of the terms like an agreement or contract between the two software products regarding the data exchange.
This set of rules outlines how the data should be formatted (usually in JSON), what actions can be performed, and if there are any limitations or requirements to accessing and using the data.
How APIs work
Think of APIs as a layer between the client (the front end of the program you see and interact with), and the server (the backend and database operations).
When you use an app or a website, you're the client. The server is where all the heavy lifting happens, like storing data and running processes. When you interact with the app, you’re requesting data. The request is sent to the API, which retrieves the data from the server and returns it to you.
The API is a middle layer between the client and the server, which helps the client and server communicate by making sure it’s possible to send data requests and responses.
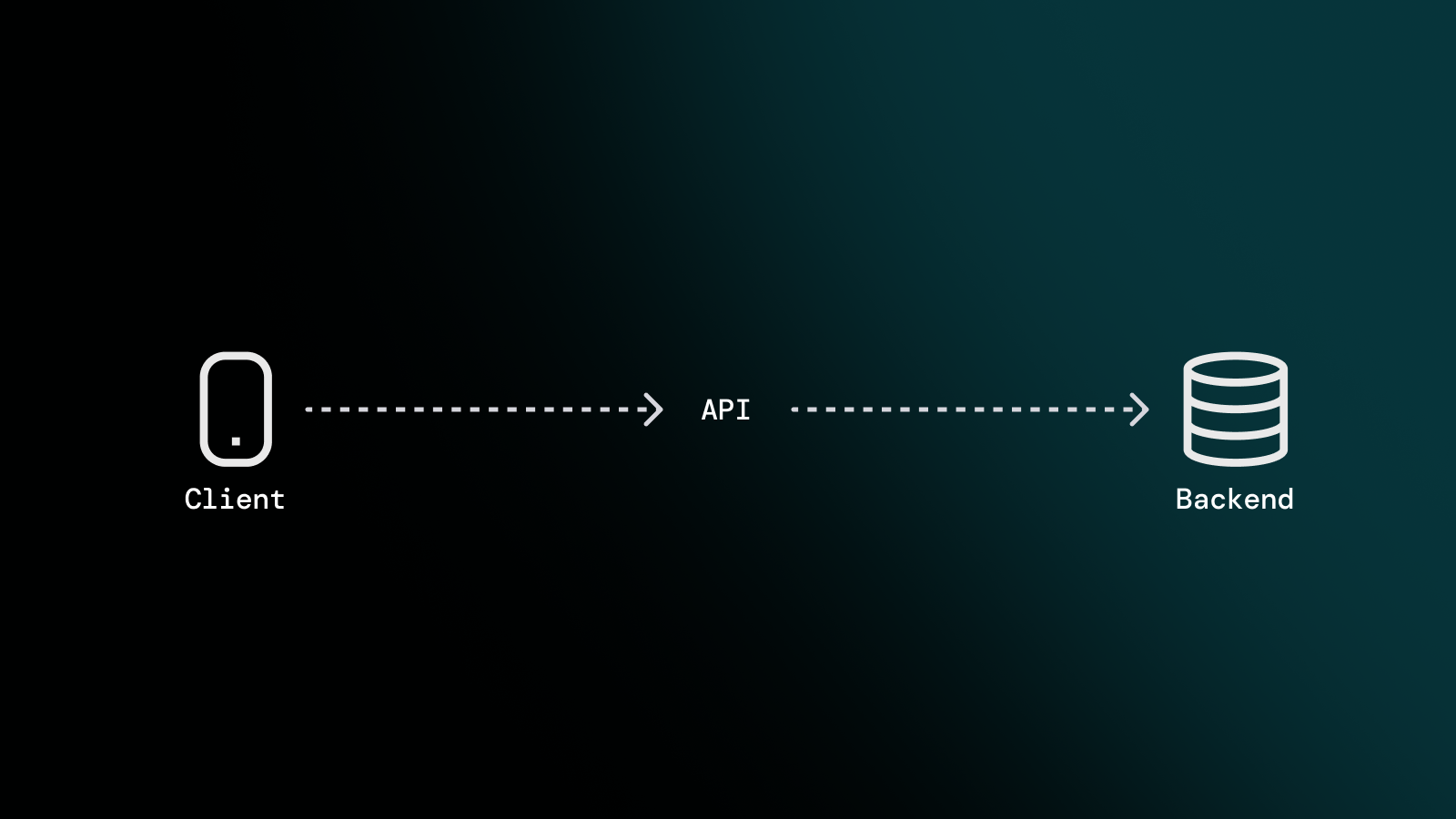
API elements
To understand how APIs work, let’s take a closer look at API elements.
API client
The API client is the program using the API to initiate the API requests. For instance, you might initiate an API request by entering a search term, clicking a button, or scrolling through a list on a web or mobile application. However, just because you initiated the API request, it doesn’t mean you’re the client, rather the app or program you’re using is the client.
API requests can also be issued in response to external events, such as a notification from another application or integration. No matter how the API request starts, the API client puts it together and sends it to the API server.
API request
An API request is the message sent by the API client from one software application to another. An API request will differ based on the type of API, but it’s made up of the following:
Endpoint: This is a dedicated URL that gives access to a specific resource in an app. The resource could be the product categories or orders. For example, in an inventory app, the /products endpoint would process all the requests related to products. The API request needs to have a designated endpoint so the API server knows what action to take or what data to retrieve.
Method: The method defines the operation that the API client would like to perform on the server. For instance, in the inventory app the method could be to retrieve all of the sold products. Every API request must include a method.
REST APIs use specific HTTP methods, such as GET, POST, PUT, PATCH, and DELETE, to perform common actions like retrieving, creating, updating, or deleting data. A GET request to the /products endpoint would tell the API server to return every product in the database.
Request headers: API request headers are key-value pairs that provide information along with the request to the server. For instance, the Authorization header provides authentication credentials, such as an API key or OAuth token, to authenticate the requester.
Request body: The request body contains important information that is needed to create, read, edit, or delete a resource (CRUD). For instance, if you’re the administrator of your inventory app and you want to add a new product, the request body might include the product’s name, brand, and price. The API decides how this information should be organized like JSON or XML.
Parameters: Parameters are like the notes you attach to a request that give specific instructions to the API on what to do. These notes can be added to the URL or included in the request body. For instance, the /products endpoint of an inventory app might accept a “brand” parameter to the request. The API then uses this information to find and return products matching that specific brand.
API server
Once the API client gathers the request, the request is sent to the relevant endpoint on the API server for processing. The API server handles authentication, data validation, data manipulation, and retrieval from a database and returning the correct response to the client.
API response
An API response is the data or information that is sent back from the server to the client after the API request. It typically includes the results of the request or if the action was successfully completed. API responses normally include:
Status codes: Status codes represent the status of the client’s request, whether it was successful, it failed, or there’s an error.
Response headers: Response headers are similar to request headers, except they provide additional information about the server’s response.
Body: The response body contains the data or content that is returned by the API server in response to the client’s request. Response bodies will vary depending on the request, but they normally include structured data objects (JSON) that represent the requested resources.
Types of APIs
There are different types of APIs and ways to categorize them. There are six primary types of APIs: Open APIs, Public APIs, Partner APIs, Private APIs, Composite APIs, and Unified APIs. We can categorize examples of APIs in two ways: by who has access to them (public, private, and partner) or by their use cases, such as database, operating system web APIs, etc.
APIs by availability
Public APIs: Public APIs provide public access to an organization's data, functionality, or services, and you can integrate the API into their own applications. Glide’s APIs are public APIs that can be used to connect your Glide apps to other tools without having to build extra functionalities or write lines of code.
Private APIs: Private APIs are also known as internal APIs, which are used to connect different software components within a single organization. Private APIs are not available for third-party use. For instance, a social media app like X might have a private API that handles the login workflow, another private API that handles the timeline, and another private API that enables communication between users.
Partner APIs: Partner APIs enable two or more business partner companies to share data to collaborate on a project. Private APIs are not available to the general public and require complex authentication mechanisms to ensure no one else can access them.
APIs by use case
Database APIs: Database APIs enable communication between an application and a database management system (DBMS). Developers work with databases by writing queries to access data, change tables, etc. Some database APIs allow users to write unified queries for different databases, like MongoDB, PostgreSQL, and MySQL.
Operating systems APIs. Operating system APIs define how applications can use the specific operating system. Every OS has its own set of APIs. For instance, Apple provides API references for macOS and iOS in its developer documentation.
Web APIs. Web APIs are the most common APIs. They enable web-based systems to share data and functions. Web APIs work with the client-server setup commonly used on the web. Web APIs use HTTP to send requests from web apps and get responses from servers.
APIs by architectural style
You can also categorize APIs according to their architectural style. The most frequently used architectural styles are:
REST: REST is the most popular API architecture for transferring data over the internet. Resources are accessible via endpoints, and operations are performed on those resources with standard HTTP methods such as GET, POST, PUT, and DELETE.
SOAP: SOAP stands for Simple Object Access Protocol. SOAP APIs use XML to transfer highly structured messages between a client and server.
GraphQL: GraphQL is an open-source query language that allows clients to interact with a single API endpoint to retrieve the exact data they need, without chaining multiple requests together.
gRPC: RPC stands for Remote Procedure Call, and gRPC APIs were originated by Google. With gRPC APIs, a client can call on a server as if it is part of its own program, which eliminates dealing with the complexities of network communication and API calls,
APIs in action
APIs work through two elements: endpoints and calls. Let's look at how those work together.
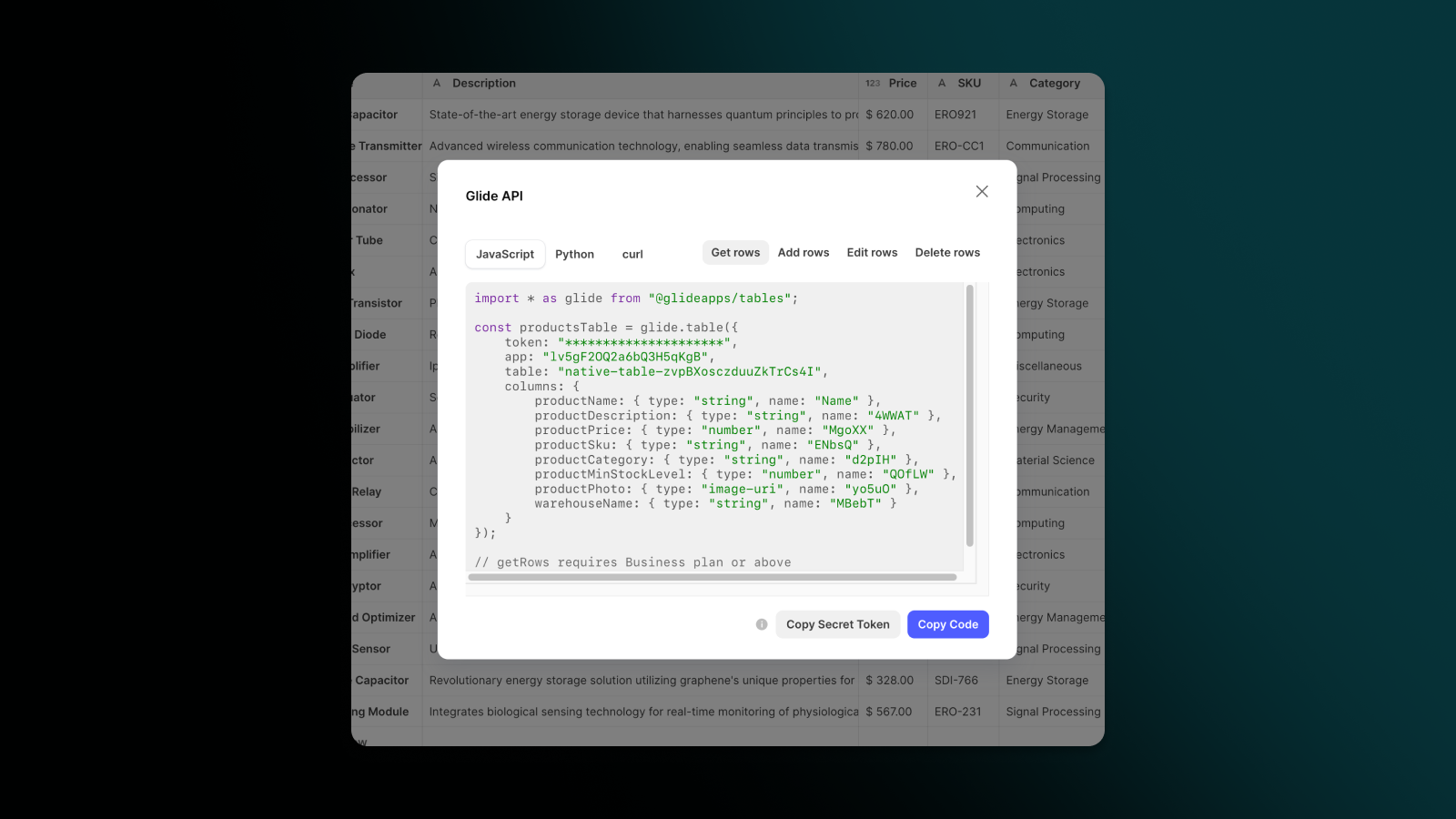
What is an API endpoint
API endpoints are touchpoints in the API communication loop. It’s a URL that serves as a gateway for sending requests and receiving responses. Each endpoint is designed to perform a specific task, such as retrieving, updating, or deleting data.
It's important to be able to identify the endpoint because even though you're not writing code, you’ll still need to specify which API endpoint you want to interact with for your integration. Being able to identify the endpoint in API documentation ensures that you can effectively use the API.
Using Glide’s Call API, you’ll need to specify the endpoint when setting up the API call. This endpoint usually corresponds to the URL of the specific resource within the external application you're connecting to.
What is an API call
An API call is a request that is sent from one software application to another using an API. An API call includes specific instructions or parameters relating to what data is needed or what action should be performed within the application. When you perform an API call, you make a request to an HTTP endpoint.
With Glide’s Call API, you can call any API and return data directly into your app, fast. You can retrieve data, create records, and even delete records.
Let’s say you're building a customer portal using Glide, and you want to display information about your customers from an external app like Salesforce. You can use the Call API feature to retrieve customer data and display it in your app.
For example, you could create a screen/column in your Glide app called "Customer Information" where your team can view customer information. You can then use the Call API feature to make an API call to Salesforce that stores information on your customers.
Using Glide’s Call API and Salesforce allows your team to access up-to-date customer data directly from your customer portal in Glide, without needing to switch between different apps. As a result, your team can efficiently manage customer relationships and provide personalized experiences.
When you integrate Salesforce data into your Glide app using the Call API feature, you centralize your customer information without the need for additional Salesforce seats. You'll save money on purchasing multiple licenses for Salesforce, as your team can access the data directly from your customer portal in real time.
This integration also helps ensure greater compliance. Since only you, the administrator, will have access to Salesforce, you can maintain strict control over who can edit data in Salesforce. Unauthorized staff members won’t have access to Salesforce data as only authorized members can make changes, enhancing compliance and data security.
How are APIs used
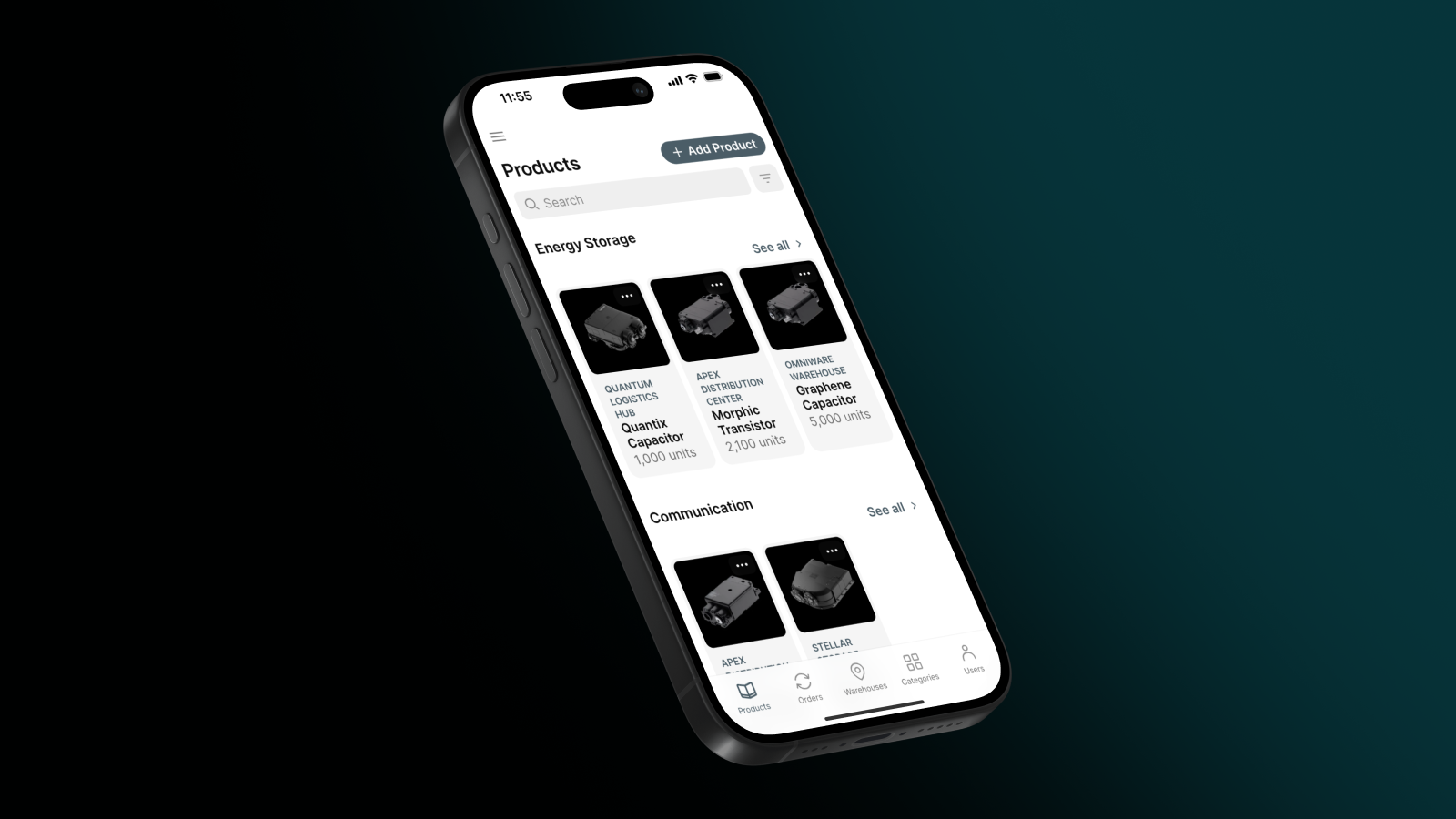
APIs are used to enable frictionless communication between different applications, allowing you to exchange data, automate processes, and enhance your current tool’s capabilities. Using APIs, you can integrate a wide range of tools to customize your existing software solutions to meet your unique business needs. APIs enable:
Data Extraction and Sharing: APIs allow you to access and share data from a wide range of sources, such as SQL databases or CRM systems. When you have access to more sources of data, you’re able to unlock hidden insights such as customer behavior patterns and sales trends. These hidden insights will lead you to make better-informed decisions that will bring value to your business or organization.
Integrations: APIs play a crucial role in integrating different software systems. When you integrate all your tools and centralize your data touchpoints, you’ll have better accuracy of data.
For instance, your Glide app can be integrated with platforms like Pipedrive and QuickBooks even though there isn’t an existing native integration. Use an API to sync your Pipedrive and QuickBooks data with your business report app to break down data silos by enabling both sales and accounting teams to access the financial data in real time on the app. Sales orders, invoices, and financial transactions will be accurately updated.
Instead of holding weekly sales meetings to relay the latest sales data to the accounting team manually, they’ll be able to view it themselves. You’ll save company time and labor costs (the intern won’t have to pull the latest data!)
Automation: APIs enable you to automate repetitive tasks as the programs are able to interact with each other and perform actions without human intervention For instance, with the Call API, you can automatically update your inventory management app based on real-time sales data from Stripe.
You’ll be able to avoid manually updating your inventory and cross-referencing sales data, enabling you to focus on other areas of the business.
Customization: Oftentimes, off-the-shelf software lacks specific functionalities or is full of bloatware features that you don’t need yet are forced to pay for. APIs enable you to customize your software according to your specific needs.
Instead of clicking through multiple interfaces, you can have all your necessary functionalities in one place. Let's say you're using Glide to create custom work order software for your maintenance team. You like Glide's user-friendly interface to display your team’s pending work orders, but you also appreciate the data entry features of another app like Google Sheets.
By using Glide’s API, you can integrate your work order app with Google Sheets. When information about a work order is entered into Google Sheets, that information is automatically synced to the work order app. You’re leveraging the strengths of both platforms, enhancing your workflow management capabilities within Glide while benefiting from the ease of data entry with Google Sheets. You’re more efficient by creating specific workflows that work for you and your team.
Create your own apps and integrate them using APIs
Creating your own apps using a no code platform like Glide and integrating them with existing off-the-shelf software gives you tools that are much more tailored to your specific business.
Glide enables any business to build custom software to fit their business processes without having to use programmers or even knowing the fundamentals of coding languages like Javascript or Python.
The tools you build with Glide are progressive web applications. This means they are functional on any device since they are accessed via a web browser. This accessibility can help make your software more adaptable to different environments where a smartphone or tablet might be easier to use than a computer.
If your existing business software is inflexible or doesn’t meet the needs of your team, use an API to connect it with a custom app to create a tool that better meets all your needs.
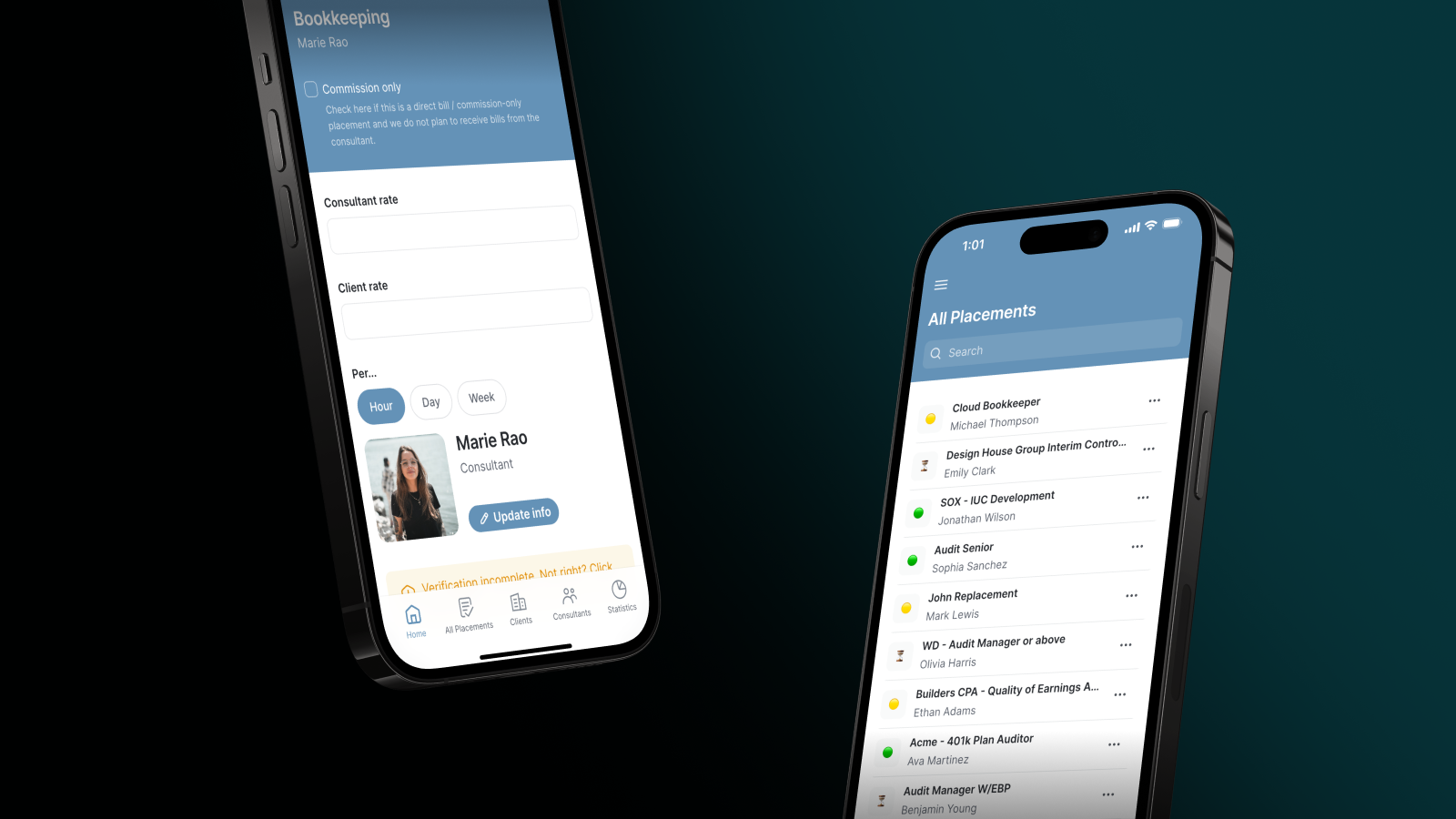
Types of business apps you can build with an API
While APIs can be used with almost any type of app, there are a few use cases that are especially powerful. An example might be an e-commerce business that is tied to a specific niche POS system. They could build a reporting app that has better data visibility and import data from their POS with an API so that management can more easily understand sales patterns.
Custom API connections can be used to create:
Advanced business intelligence apps: Integrate your business report app with your CRM to generate in-depth reports on customer interactions and sales data. Each time a customer interacts with your business through an inquiry or purchase, the interaction is synced to your business reports. You’ll be able to deduce how customer interactions directly contribute to your sales pipeline.
Customer portals: Connect your customer portal with your inventory management system to enable real-time inventory updates and leave order notes in one place. You can view and filter your top customers by searching specific inventory SKUs. Personalize customer interactions by sending automated emails reaching out to customers proactively between orders.
Employee Portals: Integrate your attendance tracking system with your employee portal to automate timekeeping and monitor employee attendance and absence reporting. This is especially helpful if your labor force is shift workers. You’ll be able to accurately record their work hours and process payroll, simplifying manual administration tasks.
Benefits of building your business software with an API
Building your business software with an API offers the following benefits:
Saves time: Building custom business software takes time. You’ll need to tackle a lot of moving parts — UX, frontend, backend, data security, and more. In Glide, you can build the software you need in a matter of hours, and with integrations, you can get your custom business software set up on the same day.
Greater view and transparency of business operations: By integrating all the different tools through APIs, you gain a comprehensive view of your business operations, you’re able to track every interaction and financial transaction, you’ll know what’s going on in each department, and you’ll be able to make better decisions with the data to back it up.
Maximizes business efficiency: 58% of employees spend their day doing “work about work", including communicating about work, searching for information, switching between apps, managing shifting priorities, and chasing status updates. Using APIs lets you automate repetitive tasks, so your team won’t spend hours a day doing tasks unrelated to their jobs.
Scalability: API-based solutions are scalable, allowing your business software to grow along with your business needs. You might start off with limited integrations, but as your business expands, you’ll incorporate new features and data sources without major disruptions or overhauls. This scalability means that your business software remains adaptable and capable of handling increased demands as your company grows.
Why are APIs important in no code?
APIs are the standard way for no code apps to communicate. They allow you to connect different apps and data sources without resorting to code. APIs expand the capabilities of the no code platforms you use, allowing you to access a wider range of features and build more sophisticated applications and workflows.
When you use APIs in combination with no code tools, you’re able to build a custom solution specific to your business while eliminating the complication of building custom apps from scratch. You end up saving time and reducing costs.
How APIs work in Glide
While Glide offers a broad range of native integrations, APIs let you create your own custom integrations with any other software your business uses. If an integration for a tool you need doesn’t already exist, you can simply create your own. This makes your Glide app much more flexible and helps you connect it to the rest of your tech stack.
Glide offers two APIs: Call API and Glide API. Call API should be used when you’re initiating from Glide, while Glide API is designed to help when initiating from outside Glide. Both offer authentification, verifying your identity with your secret API key. The API key ensures that only you can access and modify the data.
Glide API
If you’re hosting your data in Glide Tables or Big Tables you may need to access or edit your Glide data from within another service. This is what Glide API helps with. Every Glide Table or Big Table has its own API that allows you to add, edit, delete data, and export data with the get all rows method. If you’re using Big Tables, you can also query that data with SQL.
This might be used to send invoice information submitted through your Glide app into your accounting records in Quickbooks, for example.
Watch our Glide API tutorial for details on how to use it in Glide.
Call API
With the Call API integration in Glide, you can call any API you have access to and then return that data directly to your app. Call API should be used when you need to import and display data in your Glide app from another piece of software you may be using, like in the e-commerce reporting dashboard example above.
Watch our Call API tutorial for details on how to use it in Glide.
Create your own integrations and your own software today
APIs are invaluable because of the endless possibilities you can create. You’re able to combine features and capabilities from various software solutions, each performing different tasks, into a single, comprehensive solution that can meet all your needs.
Don’t be limited by off-the-shelf software options presented to you. Instead, create your own. With a powerful API like Glide’s combined with ample community resources and in-depth tutorials on YouTube, you have the power to craft exactly the tools you need, to fit precisely ro your unique needs.