When you first start your coding journey, and you look into the structure and formation of web pages, the two most popular acronyms you may come across are HTML and CSS. Well, there’s another powerful acronym quietly driving the backbone of countless web applications: JSON (pronounced Jay-son).
You may not realize when you’re already using JSON. For example, you've built a custom IT management solution for your team, and you’re keeping tabs on all of your team’s devices. JSON data is fetched from the backend database and neatly presents it to you on the front end.
JSON (JavaScript Object Notation) is a data interchange format used by different web applications to communicate with each other. While JSON isn’t a programming language you need to learn, it’s important to understand how JSON works because familiarity with it will give you a solid foundation for understanding how data is exchanged between different apps and systems.
JSON often serves as the standard data format for exchanging data between clients and servers in APIs. To dive deeper into data formats and connections like JSON, APIs, and SQL, and how they work in Glide, check out Glide’s Level 3 Certification.
Let’s begin.
The who, what, and why of JSON
JSON isn’t a programming language, so what exactly is it? It is a data interchange format. A data interchange format is a way of organizing and representing data so that it can easily be shared between different computer systems or programs. Other data interchange formats include XML and YAML.
What is a data interchange format?
A data interchange format is like a universal translator that allows you to communicate with someone regardless of the language spoken by the other person. Imagine you're building a complex application using different programming languages, like Python and Java, for different components.
Each language has its own syntax and rules, much like different spoken languages. A data interchange format is like a universal translator that allows these components written in different languages to communicate easily.
Who is JSON
In the early 2000s, Douglas Crockford uncovered JSON. JSON was already in use before, but Crockford gave it its specification (and a website) and Chip Morningstar sent the first message in 2001. Despite its youth, JSON has gained widespread adoption and has become one of the most commonly used formats for data interchange in modern web development.
"I discovered JSON. I do not claim to have invented JSON, because it already existed in nature. What I did was I found it, I named it, I described how it was useful. I don’t claim to be the first person to have discovered it; I know that there are other people who discovered it at least a year before I did. The earliest occurrence I’ve found was, there was someone at Netscape who was using JavaScript array literals for doing data communication as early as 1996, which was at least five years before I stumbled onto the idea." - Douglas Crockford, The JSON Saga, 2011
What is JSON
JSON is a text-based data format derived from JavaScript. It represents data in a key-value pair format, similar to objects structured in JavaScript. Despite being derived from JavaScript, JSON is language-independent, meaning it can be used with any programming language.
JSON has become a popular choice for transmitting data in APIs, configuration files, and exchanging data between client-server applications due to its lightweight and efficient nature.
Let’s take a look at an example of how user data is stored using JSON in a customer portal app.
{
"id": "456890356",
"name": "John Doe",
"email": "john@example.com",
"subscription": {
"plan": "Premium",
"status": "Active",
"expiry_date": "2024-12-31"
},
"billing": {
"address": "123 Main St",
"city": "New York",
"country": "USA",
"payment_method": "Credit Card"
},
"activity": {
"last_login": "2024-02-21T12:30:00Z",
"sessions": 25,
"average_session_duration": "00:45:00"
}
}
That JSON data looks like this in an app:
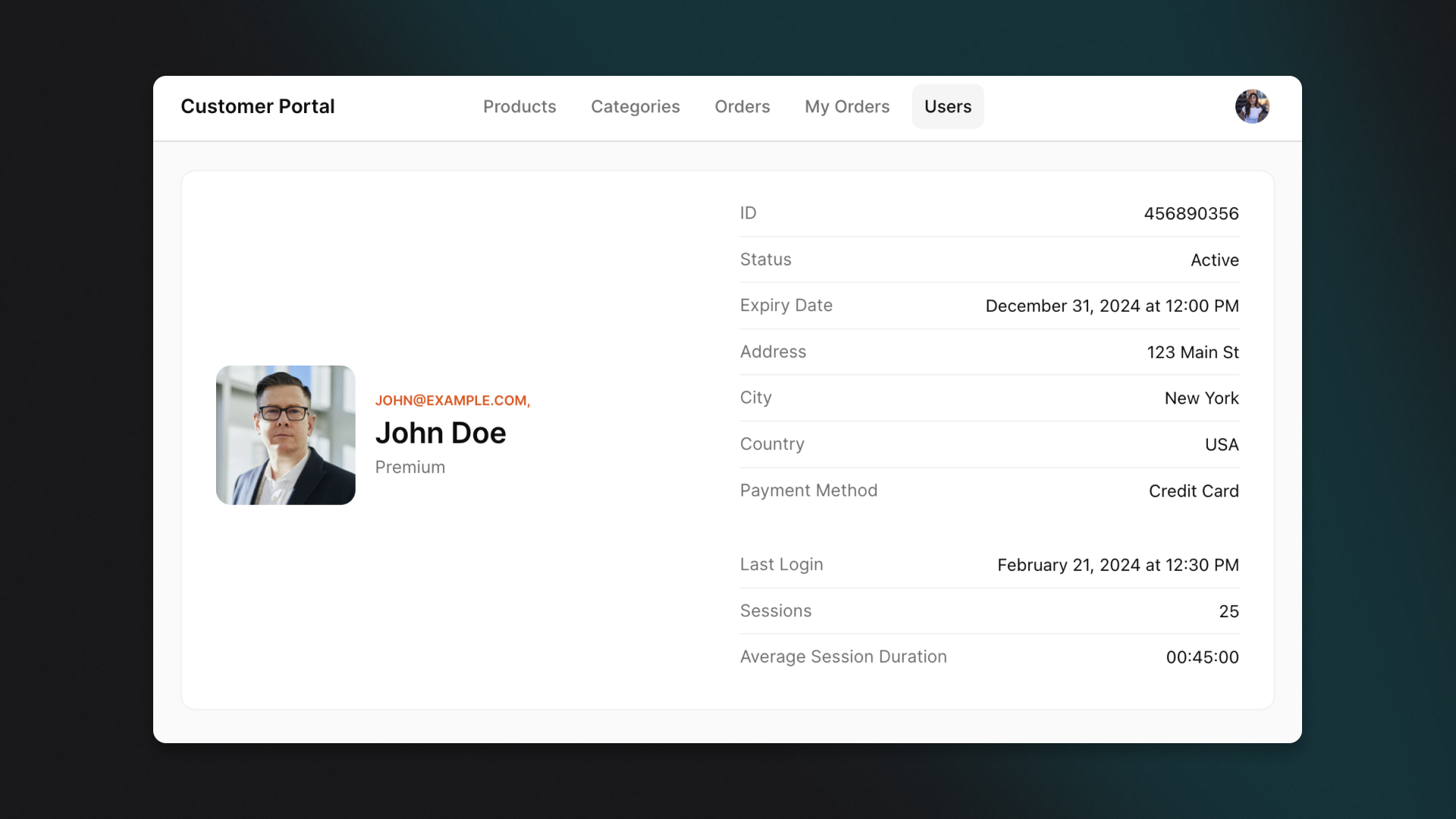
In the JSON example above, the customer is based in New York, with a premium plan, pays by credit card, and last logged into the platform in February. This user data would typically be stored in a database, such as MongoDB, MySQL, PostgreSQL, or any other database management system (DBMS).
Why JSON
JSON plays a major role in modern code architecture. JSON makes building software faster and more flexible by providing a standardized format for representing data. It pulls data from multiple sources into a package you can use repeatedly.
As APIs are becoming increasingly popular for connecting apps, JSON's role becomes even more significant, serving as the preferred format for data transfers between different systems and platforms.
JSON also offers the following advantages:
Readable: JSON uses a straightforward syntax, making it readable. Even if you've never come across JSON before, the key-value format enables you to understand what's in the data payload. JSON’s readability makes it easy to understand the data structures and makes troubleshooting and debugging easier. Once you get the hang of JSON data, it can be easy to spot any unexpected values or missing properties.
Lightweight: JSON is lightweight compared to other data interchange formats. JSON files are small and require less storage space and bandwidth when transmitting over networks. The small size of files means data is transmitted and processed fast, so apps that use JSON for data interchange can respond quickly to user interactions.
Easy to parse and generate: JSON parsers and generators are tools that simplify reading and creating JSON data in software programs. This makes tasks like converting JSON data into a format that a program can understand or creating JSON from objects or arrays simple and fast.
By using JSON.parse, you can easily convert JSON data into a format your program understands. Likewise, JSON.stringify allows you to convert objects or arrays into JSON format with ease. JSON parsers and generators simplify data interchange in software applications. In your Glide app, there’s a built-in column to parse your JSON data.
Language independence: As JSON is language-independent, it can be parsed and generated by any language that supports JSON parsing. This flexibility allows for frictionless integration with various programming languages and platforms.
JSON does have a few disadvantages. It does not enforce a schema, which means there are no automatic checks to make sure the data is organized correctly or in the right format. This means that you’ll have to ensure that the data is formatted correctly manually.
Formatting your data correctly can be overwhelming if you’re just getting started with JSON, but you can use Glide’s AI text to JSON feature to help ensure that your JSON data is formatted correctly.
Additionally, JSON does not support comments within the data, unlike XML and YAML. This can be challenging as you can’t document or annotate JSON documents with any contextual information. To combat this, you can create external documentation with your comments and annotations.
Nonetheless, JSON is widely used by developers, software engineers, and no code enthusiasts across various industries due to its simplicity and efficiency. JSON is highly accessible to a broad audience and you can use JSON to expand the capabilities of your Glide apps. You can connect your Glide app with virtually any platform using Glide’s API.
You can easily integrate real-time data from external platforms like X or Facebook and display the data in your Glide app. Glide’s API will fetch the data in JSON format, parse the data, and display it in your app.
JSON Syntax
JSON’s syntax is quite simple and follows a specific set of rules. JSON supports six data types:
Strings: JSON strings refer to text and are enclosed in double quotes.
Numbers: These are just regular numbers, which can either be negative or positive, and decimal point numbers
Booleans: These are logical representations of whether the statement is true or false.
Arrays: These are ordered lists of values that are enclosed in square brackets [ ].
Objects: JSON objects are unordered collections of key-value pairs, enclosed in curly braces { }. Each value is separated by a comma.
Null: represents an empty value.
Let’s take a look at a JSON object for a CRM app and identify each part of the syntax.
{
"company": {
"name": "The Corporation",
"industry": "Technology",
"location": "New York",
"employees": 5,
"hasCRM": true,
"departments": ["Sales", "Marketing", "Customer Support"],
"features": ["Contact Management", "Sales Pipeline", "Reporting"],
"customers": [
{
"name": "ABC Finance",
"industry": "Finance",
"contact": {
"name": "John Doe",
"email": "john@example.com",
"phone": "+1234567890"
},
"status": "Active"
},
{
"name": "ABC Inc",
"industry": "Retail",
"contact": {
"name": "Jane Smith",
"email": "jane@example.com",
"phone": "+1987654321"
},
"status": "Inactive"
}
]
},
"employees": [
{
"name": "Harry Parker",
"department": "Sales",
"role": "Sales Representative",
"isManager": false
},
{
"name": "Megan Johnson",
"department": "Marketing",
"role": "Technical Educator",
"isManager": false
},
{
"name": "Charles Brown",
"department": "Customer Support",
"role": "Customer Support Agent",
"isManager": false
},
{
"name": "Dave Jones",
"department": "Sales",
"role": "Sales Representative",
"isManager": false
},
{
"name": "Emma Davis",
"department": "Sales",
"role": "Sales Manager",
"isManager": true
}
]
}
In-app that JSON data might be displayed like this:
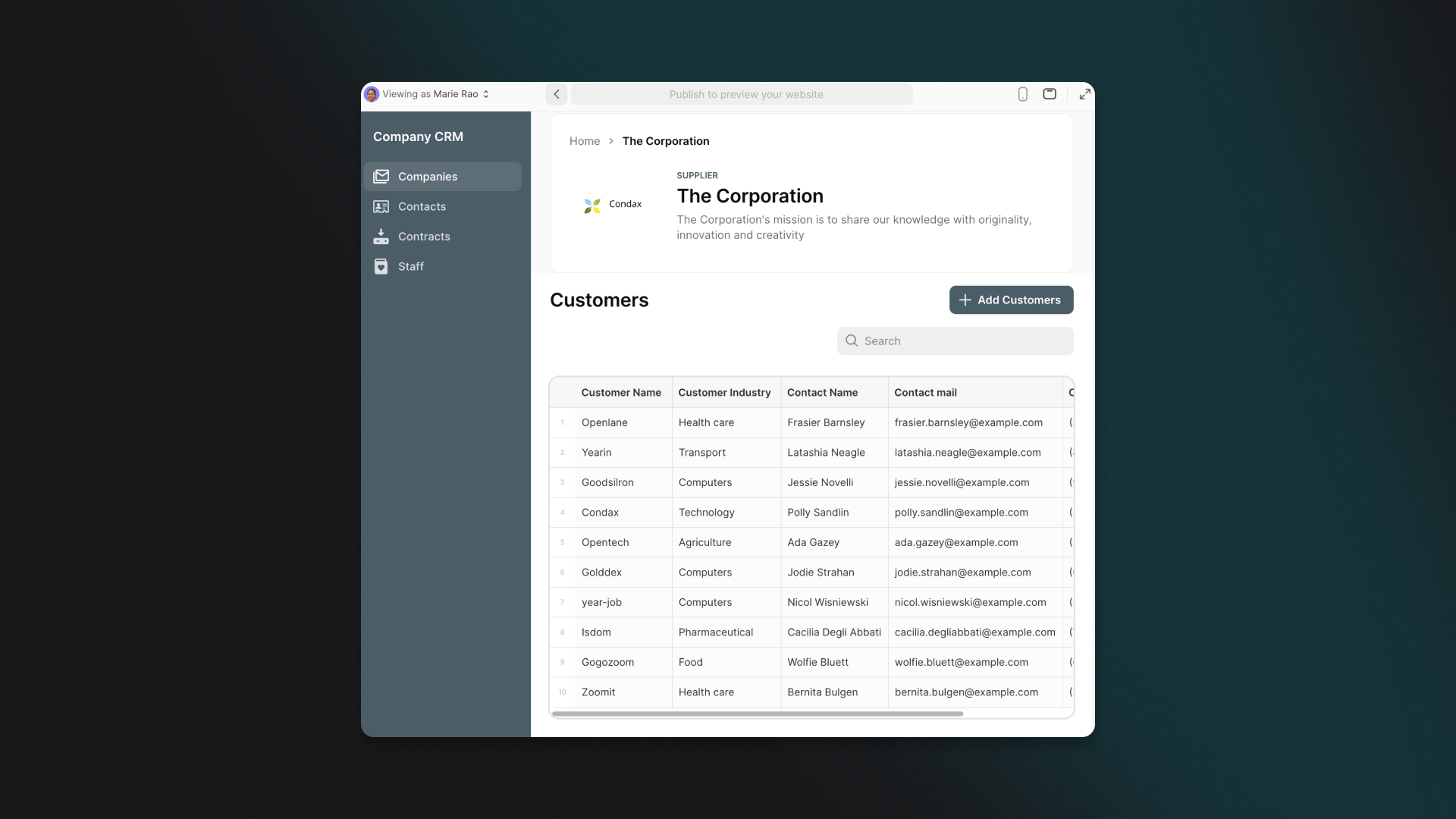
Strings: The strings are the text enclosed in the double quotes, which are the name, phone number, industry, location, email, status, and role.
Numbers: The number is the number of employees. In JSON, phone numbers are represented as strings rather than numbers.
Booleans: The "hasCRM" property is a boolean value, which is true, and the "isManager" property within each employee object is also a boolean value, indicating whether the employee is a manager or not.
Arrays: The arrays are departments, features, customers, and employees. There is a list with the values.
Objects: The entire structure is an object, enclosed in curly braces { }, representing the CRM app.
Null: In this specific object, there are no null values.
With Glide you can use AI text to JSON to format your text to JSON, but it’s still good to understand the syntax.
How is JSON used?
JSON is mainly used as a data interchange between a server (where the data is stored and managed) and a client (web browsers or mobile apps) and between different parts of an application. Every time information needs to be sent from one place to another within a software program (backend to frontend) or between different programs, JSON helps to package and transmit that information in a format that both sides can understand.
JSON is also used in:
API Responses: JSON is widely used in API responses, including Glide's Call API. When a client makes a request to an API endpoint, the server responds with JSON-formatted data. You can use the Call API to check the status of a ticket, place an order, or delete a product from a database.
Data Storage: JSON is used for storing structured data, particularly in NoSQL databases. As JSON documents are flexible and schema-less, they’re suitable for storing different JSON data types.
Data Interchange: JSON serves as a standardized format for exchanging data between different parts of an application. This could involve communication between frontend and backend components or between different microservices within a larger system.
Configuring Files: JSON is used for configuring files in software applications. JSON is used to set up and manage settings, options, and preferences. However, it does have limitations as a configuration format, as it doesn’t allow comments and annotations.
Ways to interact with JSON data in apps
Data parsing: Apps parse JSON data to extract the relevant data and use it within the app. This also includes converting JSON data into a format the app can understand and work with.
API calls: Requests from a client to a server, and the receiving responses consist of JSON-formatted data. The JSON formatted data is easily understood and processed by both the client and server, allowing the efficient exchange of data and information.
Serialization: Serialization is the process of converting data structures or objects in a programming language or text into JSON so that it can be easily understood and processed by other systems.
These methods are crucial in app development, supporting everything from parsing and converting JSON data to ensuring efficient communication through API calls and serialization. Ultimately, JSON data in apps enables the frictionless transmission and exchange of information between different systems, which ensures apps work in the way they were designed to work.
How to use JSON at work
Most people don’t realize when they’re using JSON, especially at work. JSON has many use cases in workplace apps across tools and platforms, particularly apps that require data and information exchange.
API calls in apps: JSON is the standard data format for API requests and responses due to its lightweight and easy-to-parse nature. Developers often use JSON to handle API responses and build APIs.
BI tools: Business Intelligence (BI) tools like reports and dashboards often use JSON to organize and represent data in reports and dashboards. JSON data is fetched from databases and transformed into visualizations like charts and graphs. BI platforms also provide features to parse and manipulate JSON data, allowing you to customize reports and analyze information according to their requirements.
CRM systems: As JSON is a way to store and manage data efficiently, CRM systems represent user data as JSON objects. If the CRM system integrates with an external API, this involves exchanging JSON data. JSON-based data interchange enables real-time updates and synchronization of customer records.
Project management tools: Similar to how JSON works in CRM systems, in project management tools, JSON is used to store project-related data. Integrations between your project management tool and external apps also exchange JSON data.
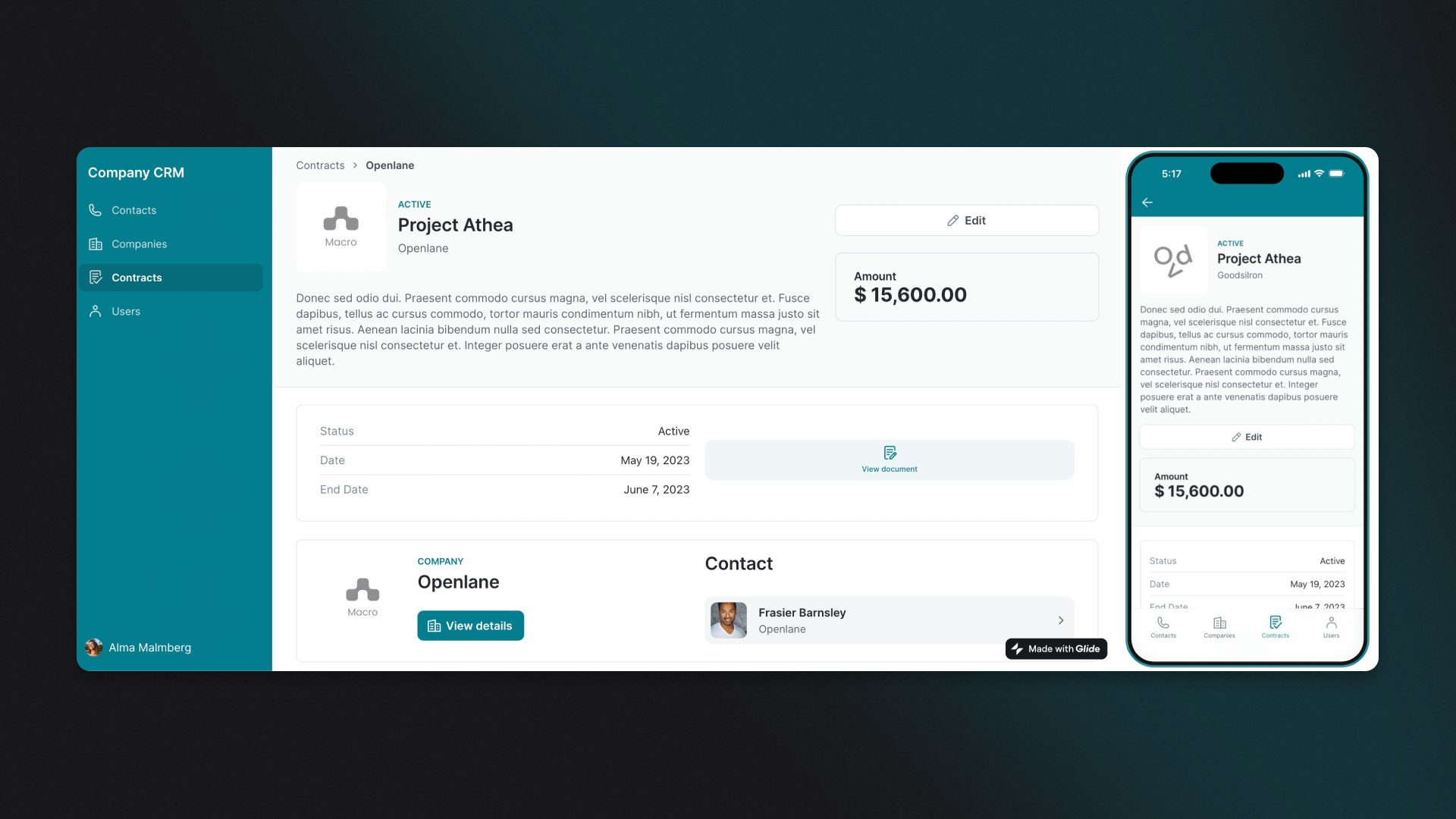
Why create a custom app with JSON data
A custom app powered by JSON data can help you simplify workflows, enhance productivity, and improve overall efficiency. For example, you can build a custom BI app that automatically enters and updates customer data whenever you get a new lead. This frees up valuable time for employees to focus on other tasks rather than data entry and reporting.
Creating a custom app with JSON has the following advantages:
Customization
When you build an app and include custom JSON data, you can easily organize and manage the information in your app the way you want.
You have the freedom to change and make customizations based on what you want and not using the parameters and options set out in standard software apps. Let’s say you’re building an inventory app for your grocery store. With custom JSON data, you can easily add new categories like "Gluten-Free" or "Vegan".
Traditional inventory apps might not give you the flexibility to add new categories to their pre-assigned categories. But using JSON, all you have to do is update the JSON data to include the inventory that fits this criteria. You can add new fields like "isGlutenFree" to each item entry (with the Boolean true/false) to filter out your gluten-free inventory items.
Without custom JSON data, adding new categories or features will require complex coding changes.
Personalization
Building an app with custom data enables you to personalize the user experience or workflow triggers in your app. Let’s consider an incident and accident app used by a construction company to manage workplace accidents. You can personalize your app in the following ways with custom JSON data:
Automatically assign employees based on employee profiles and training records: The app can store information about each employee’s role, training and certifications. This data can be used to assign the appropriate person to respond to the incident.
Tailor response plans: Based on the nature of the incident and the person involved, the app can automatically generate response plans that outline the specific actions that should be taken.
Analyze past data and spot trends: Custom JSON data can also make analysis of historical incidents easier. By storing historical data, the app can identify patterns and trends of popular incidents.
Scalability
Using custom JSON data enables you to structure and modify your app’s data as needed. When you build an app on the back of custom JSON data, you can easily extend your existing data schema to include or remove data that is relevant to the business.
For example, if you have a specific custom JSON structure for your app, you can easily introduce or remove fields to the JSON data structure. However, if you’re using an app with a predefined data structure, adding or removing fields consists of complex actions like making changes to the app’s codebase and potentially migrating data, which requires a specialized skill set.
Overall, creating a custom app with JSON data gives you the flexibility to structure your app in the way you want it. You’re able to create an app specific to your unique business needs, and you’re able to make high-level customizations without having to write complex lines of code.
How JSON works in Glide
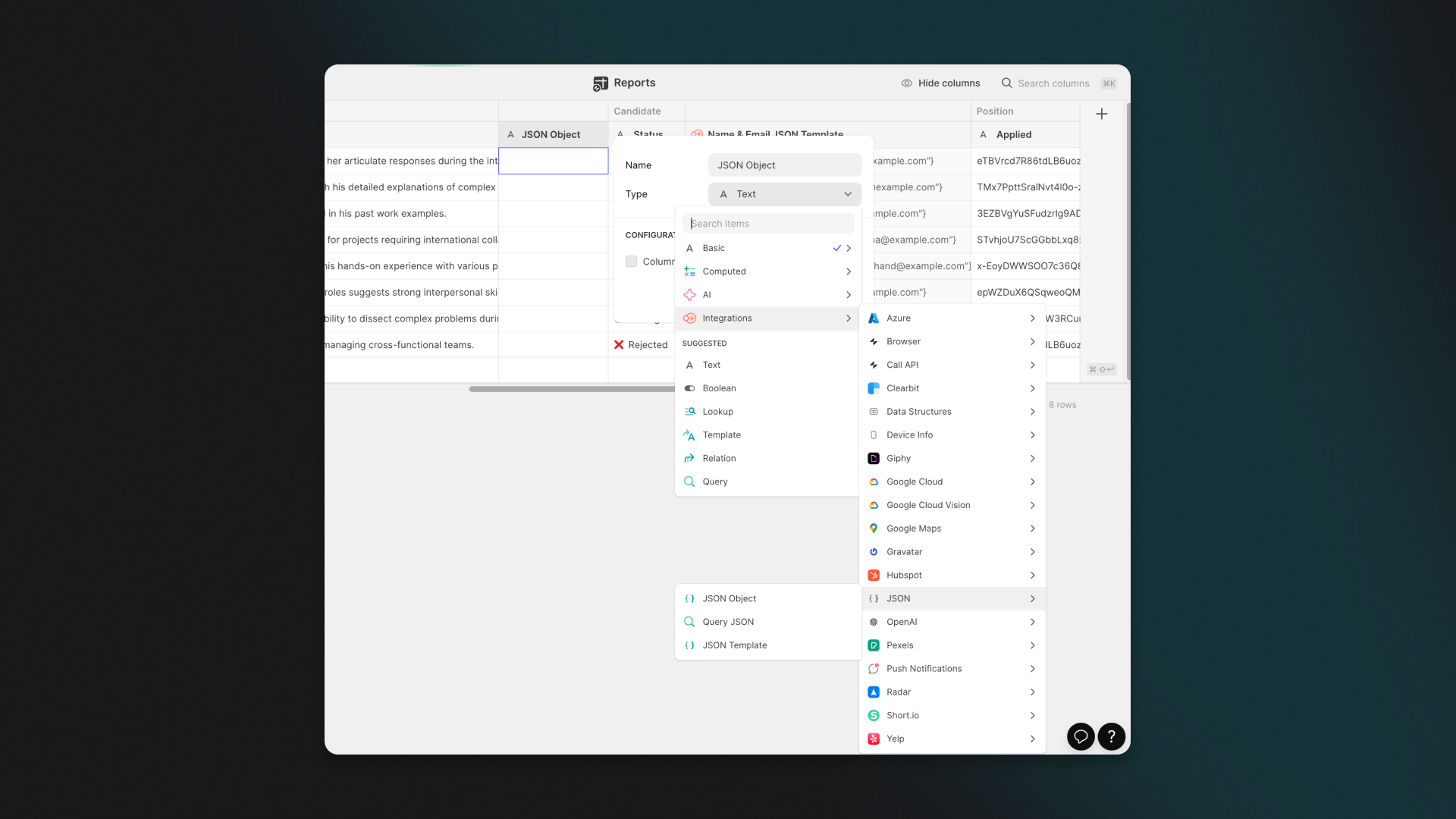
In Glide, JSON serves as a crucial tool for both sending data out to other services and receiving data from external sources. What this means is that you can send data from your Glide app to other platforms and vice versa.
JSON data is fetched from Glide’s API. The JSON response is then parsed to extract relevant information, which is transmitted to the UI components you see on your app. Simply speaking, JSON can be used to structure and transmit data between different components within your Glide app.
Using JSON in Glide enables you to develop and deliver a more personalized and tailored solution to your needs that traditional apps won’t be able to give you. You can start with templates, or build an app from scratch following the tutorials in Docs.
When you use tailored software solutions for your business needs, you’ll be able to create more value for your customers and team by focusing on more strategic initiatives rather than trying to make rigid solutions adaptable to your needs.
Build a no code app from JSON data
A no code app built from JSON data can bring invaluable benefits to your business. You’ll be able to create a tailor-made app specific to your business needs without the need to write complex lines of code if you want to make any modifications. Making modifications to existing code can be a daunting task, it’s expensive, time-consuming, and requires specialized skills.
Building an app from JSON data will enable you to make modifications in a few clicks.
JSON is a valuable skill to learn, especially as the software world becomes more hyperconnected and APIs become increasingly popular. Learning about the basics of JSON will help you understand how data is exchanged between apps.
To learn more about the principles of building apps in Glide with JSON, check out the lessons in Glide University or take the Level 3 Glide Certification course.